
In order to do this, you can use the gauss() function, which accepts both the mean and the standard deviation of the distribution. The random library also allows you to select a random value that follows a normal Gaussian distribution. # Returns: Generate a Random (Normal) Gaussian Distribution in Python Random_list = random.sample(range(0, 16), k=5) So, say you wanted to select five values without substitution between 0 and 15, you could write: # Generate a list of random integers without substitution The function expects a list of values and the number of values to select. In order to do this, you can use the random.sample() function. This means that you can select a number once, and only once.
RANDOM DATA GENERATOR PYTHON HOW TO
In this section, you’ll learn how to generate a list of random integers without substitution. # Returns: Generate a List of Random Integers without Substitution Let’s see how we can create a list of 5 random integers between 50 and 100: # Generate a list of random integers in Python To do this, we’ll create a list comprehension that calls the random.randint() function multiple times. We can apply the same approach to generate a list of random integers. # Returns: Generate a List of Random Integers We can apply the same approach to create a list of random floats between two given numbers: # Generate a list of random floats between 10 and 30 The list comprehension repeats calling the.We instantiated a new list, random_list which holds a list comprehension.In the examples below, we’ll use a Python list comprehension to generate two lists: one with random floats between 0 and 1 and the other with random floats between two specified numbers: # Generate a list of random floats between 0 and 1 We can use either a for loop or a list comprehension. In order to generate a list of random floats, we can simply call the. By the end of this, you’ll learn how to select a list of random floats, random integers, and random integers without repetition. Because this can be done in different ways, we’ll split this section into multiple parts. In this section, you’ll learn how to generate a list of random numbers in Python.
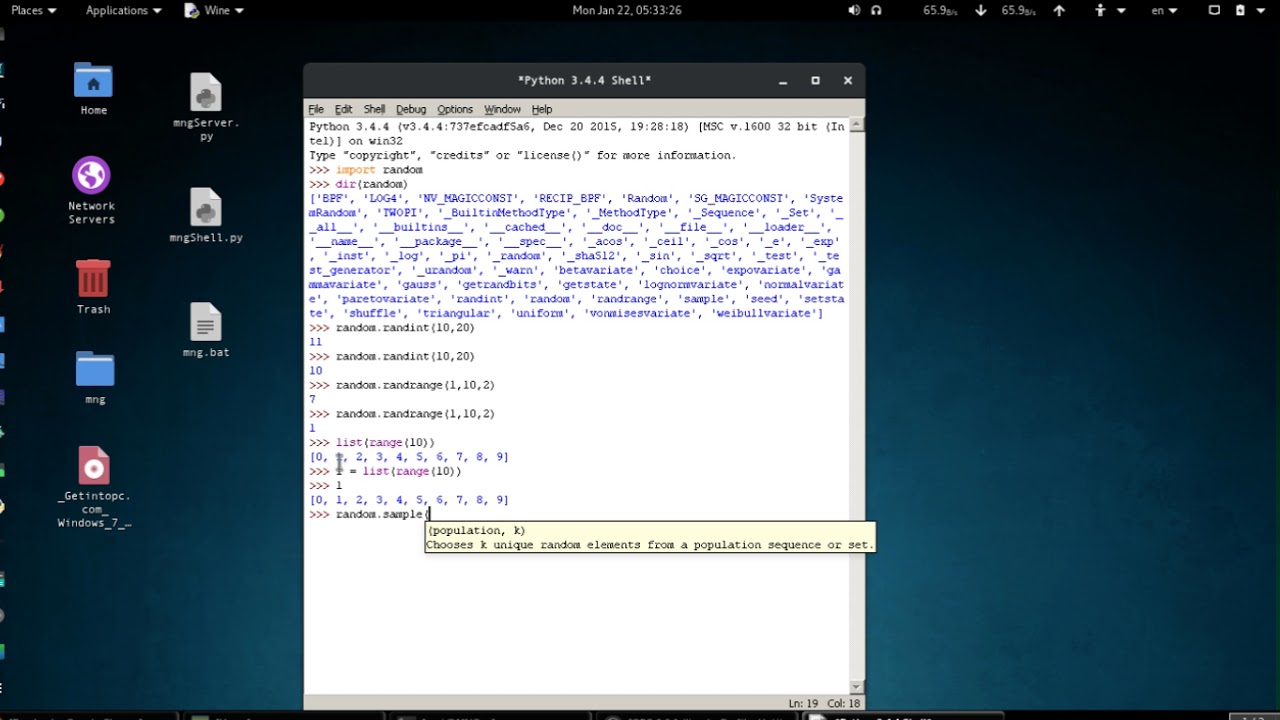
Generate a List of Random Numbers in Python In order to include it, simply add 1 to the value, such as random.randrange(0, 101, 3). The important piece to note here is that the upper limit number is not included in the selection. Let’s see how this works: # Choosing a Random Value from a Range This means that say you wanted to choose a random number between, say, 0 and 100, but only in multiples of 3.įor this, you can use the randrange() function. In this section, you’ll learn how to generate random numbers between two values that increase at particular steps. # Returns: -24 Generate Random Numbers Between Two Values at Particular Steps in Python Let’s repeat this example by picking a random integer between -100 and 100: # Generating a random integer between two values However, if we wanted to generate a random number between two values, we can simply specify a different value as the starting point (including negative values). In the example above, we used 0 as the starting point. # Returns: 6 Generate Random Numbers Between Two Values in Python Let’s see how we can generate a random integer in Python: # Generating a random integer in Python

For this, you can use the randint() function, which accepts two parameters:

The random library makes it equally easy to generate random integer values in Python. In the next section, you’ll learn how to generate a random integer in Python. You can see that the random number that’s returned is between (and can include) the boundary numbers.

Let’s see how this works: # Generating a random float between two numbers However, there may be times you want to generate a random float between any two values. While the random() function generates a random float between 0 and 1. Generate a Random Float Between 2 Numbers The random() function is used to generate a random float between 0 and 1. # Generate a random float between 0 and 1 Let’s first see how to create a random floating point between 0 and 1. The library makes it incredibly easy to generate random numbers.
RANDOM DATA GENERATOR PYTHON INSTALL
This means that you don’t need to install any additional libraries. Python comes with a package, random, built in. Generate Random Floating Point Value in Python Create Reproducible Random Numbers in Python.Generate a Random (Normal) Gaussian Distribution in Python.Generate a List of Random Numbers in Python.Generate Random Floating Point Value in Python.
